Home
About Me
Portfolio
Goodies
Contact
Blog
How To Take Control of the Night (By Adding a Dark Mode Toggle to your site with Vanilla JS)
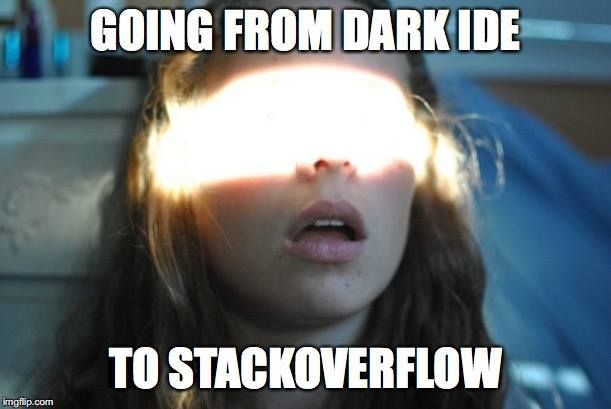
Here's a coding tip I thought y'all would find useful. Enjoy!
Have you ever been browsing a website late at night and gotten blinded by a bright white background? Yeah, me too. That's where dark mode comes in. It's a website setting that swaps the usual light color scheme for a darker, more eye-friendly one.
In this post, I'll guide you through adding a simple dark mode toggle to your website using vanilla JavaScript (just JavaScript itself, without any frameworks) and separate CSS files for light and dark mode styles.
Step 1: Setting up the HTML Structure
First, let's create a basic HTML structure for our toggle button. You can place this anywhere on your webpage that you find suitable:
<button id="dark-mode-toggle">Toggle Dark Mode</button>
This code creates a button with the ID "dark-mode-toggle" and the text "Toggle Dark Mode".
Step 2: Creating the CSS Files (light.css & dark.css)
Now, let's create two separate CSS files: light.css and dark.css. In these files, you'll define the styles for each mode. Here's a basic example:
light.css:
body {
background-color: #fff;
color: #000;
}
/* Add other styles for light mode here */
dark.css:
body {
background-color: #000;
color: #fff;
}
/* Add other styles for dark mode here */
These are just basic examples, you can customize these styles with your preferred colors and themes!
Step 3: Implementing the JavaScript Magic
Here's where the JavaScript comes in! We'll use JavaScript to listen for clicks on the toggle button and then switch between the light and dark CSS files.
Create a new JavaScript file (e.g., script.js) and add the following code:
const toggleButton = document.getElementById('dark-mode-toggle');
const lightModeCSS = 'light.css';
const darkModeCSS = 'dark.css';
let isDarkMode = false; // This variable will track the current mode
toggleButton.addEventListener('click', function() {
const currentCSS = document.head.querySelector('link[rel="stylesheet"]');
if (isDarkMode) {
currentCSS.href = lightModeCSS;
isDarkMode = false;
} else {
currentCSS.href = darkModeCSS;
isDarkMode = true;
}
});
How it Works:
- We first select the button element using its ID.
- We define the paths to the light and dark mode CSS files.
- We create a variable
isDarkMode
to track the current mode (light or dark). - We add an event listener to the button that listens for clicks.
- When the button is clicked, the code checks the current CSS file linked in the
<head>
section of the HTML. - If it's dark mode, the script switches the linked file to light.css and updates the
isDarkMode
variable. - If it's light mode, the script switches the linked file to dark.css and updates the
isDarkMode
variable.
Step 4: Tying it Up
Finally, include your JavaScript file in the HTML before the closing </body>
tag:
<script src="script.js"></script>
There we go! You've now added a working dark mode toggle to your website using vanilla JS and separate CSS files.
With a little customization, you can create a sleek and user-friendly dark mode toggle that enhances your website's browsing experience!
100+ Free Website Templates
Get fully-customizable HTML/CSS templates for free
Ads by Carbon
Subscribe, maybe?
You won't get spam. You will, however, get all my latest blog posts right into your inbox each week.